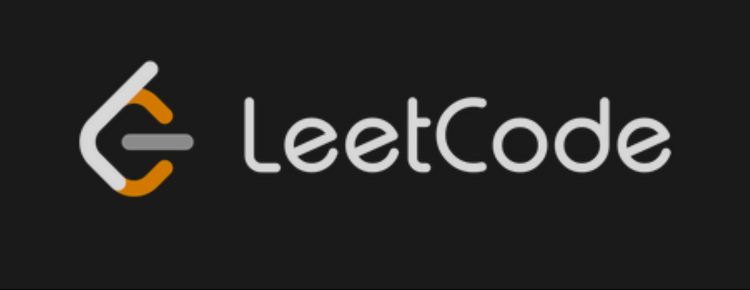
Leetcode#4_ZigZag Convention_05wk02 by SOMJANG
솜씨좋은장씨
·2020. 5. 13. 20:11
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
P A H N
A P L S I I G
Y I R
And then read line by line: "PAHNAPLSIIGYIR"
Write the code that will take a string and make this conversion given a number of rows:
string convert(string s, int numRows);
Example 1:
Input: s = "PAYPALISHIRING", numRows = 3
Output: "PAHNAPLSIIGYIR"
Example 2:
Input: s = "PAYPALISHIRING", numRows = 4
Output: "PINALSIGYAHRPI"
Explanation:
P I N
A L S I G
Y A H R
P I
Solution
class Solution:
def convert(self, s: str, numRows: int) -> str:
max_interval = 2 * (numRows - 1)
string_len = len(s)
string_list = list(s)
new_string = []
if string_len == 1 or numRows == 1:
answer = s
else:
for i in range(numRows):
index_num = i
if (i == 0) or (i == numRows - 1):
while True:
if index_num > string_len-1:
break
new_string.append(string_list[index_num])
index_num = index_num + max_interval
else:
interval_first = 2 * (numRows - 1 - i)
interval_second = max_interval - interval_first
count = 0
while True:
if index_num > string_len-1:
break
new_string.append(string_list[index_num])
if count % 2 == 0:
index_num = index_num + interval_first
elif count % 2 == 1:
index_num = index_num + interval_second
count = count + 1
answer = ''.join(new_string)
return answer
Solution 풀이
조만간 업로드 하겠습니다!
'우리가 공부한 것 들 > 알고리즘' 카테고리의 다른 글
Leetcode#3_Longest Substring Without Repeating Characters _05wk01 by SOMJANG (0) | 2020.05.11 |
---|---|
Leetcode#1_02w03 Code Review 결과 정리 (0) | 2020.02.22 |
Leetcode#1_Add Two Numbers_02w03 by SOMJANG (0) | 2020.02.20 |
Leetcode#1_Reverse Integer_02w03 by SOMJANG (0) | 2020.02.20 |